- Behavioral pattern
- The intent of this pattern is to allow an object to alter its behavior when its internal state changes. The object will appear to change its class.
- Context:- defines the interface thats of interest to its clients. It maintains an instance of a ConcreteState subclass that defines the current state.
- ConcreteState:- implements behavior associated with a state of the Context
- State:- defines an interface for encapuslating the behavior asociated with a particular state of the context.
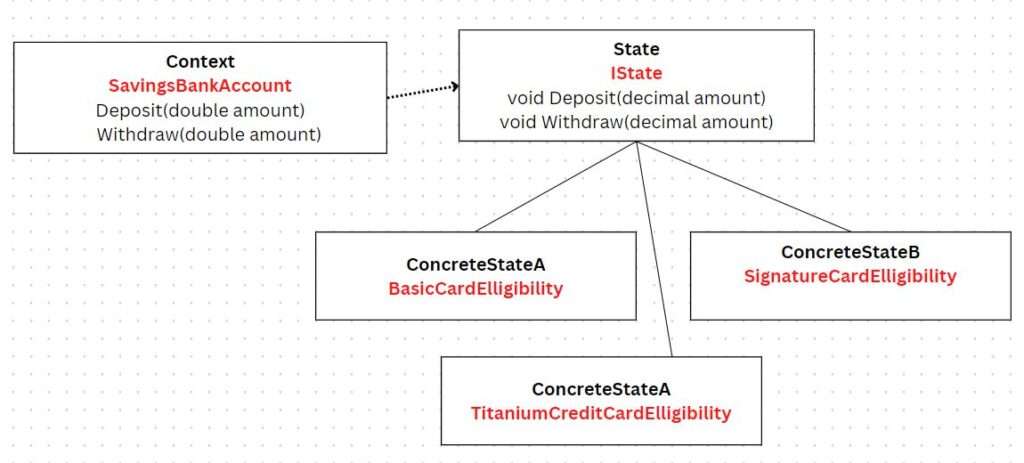
SavingsBankAccount
namespace StatePattern
{
public class SavingsBankAccount
{
private IState State { get; set; }
private double BalanceInSavingAccount { get; set; }
public SavingsBankAccount()
{
State = new BasicCardElligibility();
}
public void Deposit(double amount)
{
BalanceInSavingAccount += amount;
CheckCreditCardElligibility();
}
public void Withdraw(double amount)
{
BalanceInSavingAccount -= amount;
CheckCreditCardElligibility();
}
public void CheckCurrentCreditCardBenefits()
{
State.Facilities();
}
public void CheckCreditCardElligibility()
{
if(BalanceInSavingAccount <= 5000)
State = new BasicCardElligibility();
if (BalanceInSavingAccount > 50000 && BalanceInSavingAccount < 100000)
State = new SignatureCardElligibility();
if (BalanceInSavingAccount >= 100000)
State = new TitaniumCreditCardElligibility();
}
}
}
State
namespace StatePattern
{
public interface IState
{
void Facilities();
}
public class BasicCardElligibility : IState
{
public void Facilities()
{
Console.WriteLine("Basic Card");
Console.WriteLine("1 movie ticket free in 6 month");
}
}
public class SignatureCardElligibility : IState
{
public void Facilities()
{
Console.WriteLine("Signature Card");
Console.WriteLine("2 movie ticket free in 1 month");
}
}
public class TitaniumCreditCardElligibility : IState
{
public void Facilities()
{
Console.WriteLine("Titaninum Card");
Console.WriteLine("5 movie ticket free in 1 month");
}
}
}
PROGRAM.CS
using StatePattern;
SavingsBankAccount savingsBankAccount = new();
savingsBankAccount.Deposit(1000);
savingsBankAccount.CheckCurrentCreditCardBenefits();
Console.WriteLine("---------------------");
savingsBankAccount.Deposit(50000);
savingsBankAccount.CheckCurrentCreditCardBenefits();
Console.WriteLine("---------------------");
savingsBankAccount.Deposit(60000);
savingsBankAccount.CheckCurrentCreditCardBenefits();
Console.WriteLine("---------------------");
savingsBankAccount.Withdraw(20000);
savingsBankAccount.CheckCurrentCreditCardBenefits();
OUTPUT
Basic Card
1 movie ticket free in 6 month
———————
Signature Card
2 movie ticket free in 1 month
———————
Titaninum Card
5 movie ticket free in 1 month
———————
Signature Card
2 movie ticket free in 1 month
GITHUB