- A delegate is a type that represents reference to methods with a particular parameter list and return type
- A delegate is the glue/pipeline between
an event and event handler - Specialised class based on the MulticastDelegate base class.
- Often called as function pointer.
- Delegates allows you to reference a method and later invoke it.
- A object that knows how to call a method or group of methods
When to use Delegate
- When you need a callback.
- Commonly used in past performing background work
- When a publisher notifies that an event
has occurred, every subscriber is executed and can act on it. - Allow a method to run additional functionality or replace functionality during runtime.
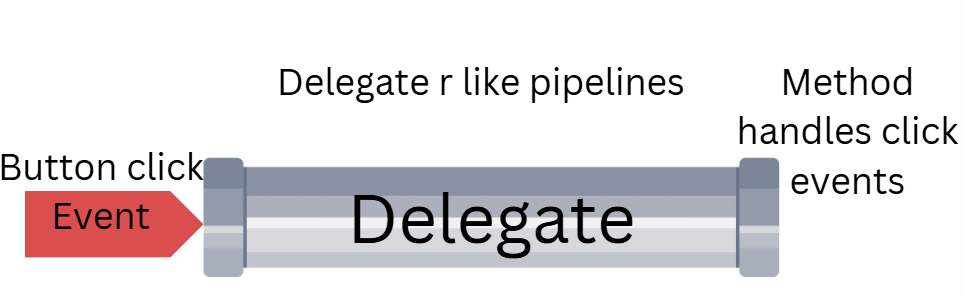
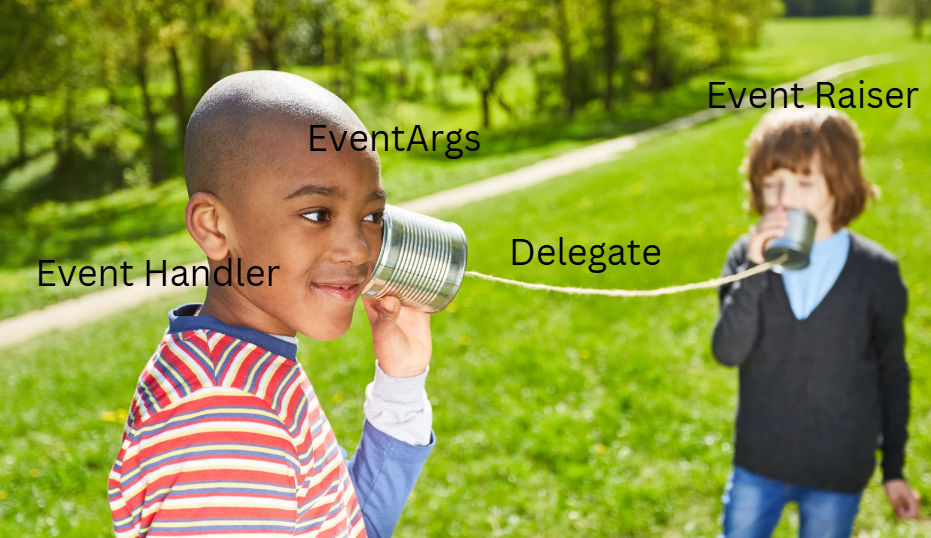
Extensibility
House house = new House();
var buildHouse = new HouseBuild();
buildHouse.PaintBlue(house);
buildHouse.SemiFurnished(house);
buildHouse.DecorateBasicInterior(house);
public class House
{
}
public class HouseBuild
{
public void PaintBlue(House house)
{
Console.WriteLine("Painting Blue Color");
}
public void DecorateBasicInterior(House house)
{
Console.WriteLine("Decorate basic interior");
}
public void SemiFurnished(House house)
{
Console.WriteLine("Semi Furnished !!");
}
}
output :-
Painting Blue Color
Semi Furnished !!
Decorate basic interior
- Now If I want to modify the HouseBuild, I dont want to touch the existing class, rather I want to modify in my own way.
- Lets use Delegate to extend it.
- The same thing can be done using interface as well.
House house = new House();
var buildHouse = new HouseBuild();
//buildHouse.PaintBlue(house);
//buildHouse.SemiFurnished(house);
//buildHouse.DecorateBasicInterior(house);
HouseHandler obj = buildHouse.DecorateBasicInterior;
//obj += buildHouse.SemiFurnished;
obj += PaintWhite;
obj += FullFurnishedHouse;
obj.Invoke(house);
static void FullFurnishedHouse(House house)
{
Console.WriteLine("This is full furnished house with all the amenities");
}
static void PaintWhite(House house)
{
Console.WriteLine("The house is painted white");
}
public delegate void HouseHandler(House house);
Output:-
Decorate basic interior
The house is painted white
This is full furnished house with all the amenities
Action Delegate to replace HouseHandler
Everything else remains same. We get the same output
Action obj = buildHouse.DecorateBasicInterior;
//obj += buildHouse.SemiFurnished;
obj += PaintWhite;
obj += FullFurnishedHouse;
obj.Invoke(house);
- Alternatively we can use interface
- How to decide whether to use interface or delegate ??
- The caller doesnt need to access other properties or methods on the object implementing the method
- A class may need more than one implementation of the method.
Delegate vs Interfaces Example
delegate void MyHouseHandler(string name);
interface IHouseHandler
{
void BuildHouse(string house);
}
class HouseUsingDelegate
{
// All four methods below can be used to implement the MyHouseHandler delegate
void BuildHouse1(string house) { }
void BuildHous2(string house) { }
void BuildHous3(string house) { }
void BuildHous4(string house) { }
}
class HouseUsingInterface : IHouseHandler
{
public void BuildHouse(string house)
{
// Only this method could be used to call an implementation through an interface
}
}