- Records are immutable by default.
- All the record members are init-only properties. Below is the screenshot.
CODE - CLASS
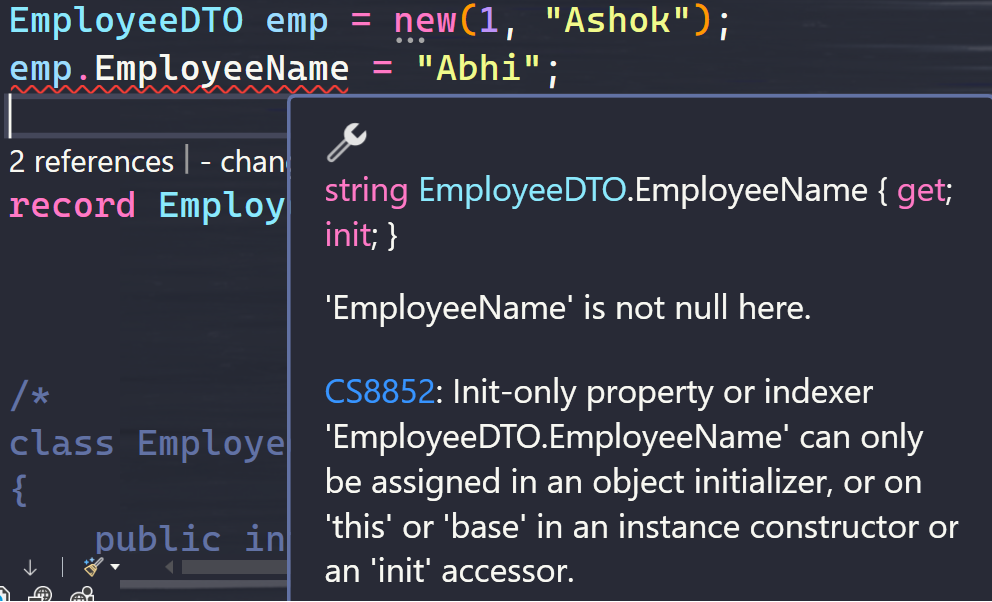
CODE - CLASS
Employee emp1 = new Employee() { Empid = 1, EmployeeName = "Rahul", Salary = 10000 };
Employee emp2 = new Employee() { Empid = 1, EmployeeName = "Rahul", Salary = 10000 };
Console.WriteLine(emp1);
Console.WriteLine(emp1 == emp2);
//class Employee
//{
// public int Empid { get; set; }
// public string EmployeeName { get; set; }
// public int Salary { get; set; }
//}
class Employee
{
public int Empid { get; set; }
public string? EmployeeName { get; set; }
public int Salary { get; set; }
public override bool Equals(object? obj)
{
if (obj is null || obj is not Employee)
return false;
var otherEmployee = (Employee)obj;
if (Empid != otherEmployee.Empid || EmployeeName != otherEmployee.EmployeeName || Salary != otherEmployee.Salary)
return false;
return true;
}
public static bool operator ==(Employee x, Employee y)
{
return x.Equals(y);
}
public static bool operator !=(Employee x, Employee y)
{
return !x.Equals(y);
}
public override string ToString()
{
return $"Personclass {{Name ={EmployeeName} and Salary = {Salary} }}";
}
}
CODE - RECORD
//1.Records are reference type like class
//2.Immutable
//you cannot change the value once they are set... so it is init only properties
//init only properties can only be set in construtor initialization or object initializer
//3.Faster than classes
//4.Value based comparision
//5.Where to use--> you can use in DTO classes..
//6.No need to ovverideToString() Method as we used to do in classes
//7.No need to ovveride Equals Object...
//8.Inherit a new record from existing record
var employee1 = new EmployeeRecord(1, "Rahul", 1000);
var employee2 = new EmployeeRecord(1, "Rahul", 1000);
//Directly pring
Console.WriteLine(employee1);
//Comparision
Console.WriteLine(employee1 == employee2);
//Inherit using with operator
var employee3 = employee2 with { EmpName = "Vinod", EmpSalary = 2000 };
Console.WriteLine(employee3);
//you cannot change existing property
//employee1.EmpSalary = 4000;
public record EmployeeRecord(int EmplId, string EmpName, int EmpSalary);
- init only property can only be assigned only in constructor or object-initializer.