- Install the below packages
- System.IdentityModel.Tokens.Jwt
- Microsoft.AspNetCore.Authentication.JwtBearer
AuthController
- This controller will be responsible to create the token.
- GenerateToken method generates the token. The token will be always unique in every subsequent calls.
using Microsoft.AspNetCore.Mvc;
using Microsoft.IdentityModel.Tokens;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
using System.Text;
namespace JWT_WebApi.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class AuthController : ControllerBase
{
private readonly Jwtsettings _jwtsettings;
public AuthController(Jwtsettings jwtsettings)
{
_jwtsettings = jwtsettings;
}
[HttpPost("token")]
public IActionResult GenerateToken()
{
var claims = new[]
{
new Claim(JwtRegisteredClaimNames.Sub,"testuser"),
new Claim(JwtRegisteredClaimNames.Jti,"testpassword"),
};
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_jwtsettings.SecretKey));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
var token = new JwtSecurityToken(
issuer: _jwtsettings.Issuer,
audience: _jwtsettings.Audience,
claims: claims,
expires: DateTime.Now.AddMinutes(_jwtsettings.ExpirtyMinutes),
signingCredentials: creds
);
return Ok(new JwtSecurityTokenHandler().WriteToken(token));
}
}
}
CompanyController
- The Get method is marked with authorize attribute.
- When you remove the authorize attribute, you can call this method without any hassle.
- With Authorize attribute if you don’t pass bearer token you get 401 unauthorized error
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Mvc;
namespace JWT_WebApi.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class CompanyController : ControllerBase
{
[HttpGet]
[Authorize]
public IActionResult Get()
{
return Ok(new { test = "This is a protected value.." });
}
}
}
Jwtsettings Model
namespace JWT_WebApi
{
public class Jwtsettings
{
public string SecretKey { get; set; }
public string Issuer { get; set; }
public string Audience { get; set; }
public int ExpirtyMinutes { get; set; }
}
}
Program.cs
- The points marked with 1,2,3 and 4 are added as part of jwt configuration
using JWT_WebApi;
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.IdentityModel.Tokens;
using System.Text;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
//1. jwt configure
var jwtSettings = new Jwtsettings();
builder.Configuration.GetSection("JwtSettings").Bind(jwtSettings);
builder.Services.AddSingleton(jwtSettings);
//2. jwt AddAuthentication
builder.Services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
}).AddJwtBearer(options => {
options.TokenValidationParameters = new Microsoft.IdentityModel.Tokens.TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = jwtSettings.Issuer,
ValidAudience = jwtSettings.Audience,
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(jwtSettings.SecretKey))
};
});
//3....jwt
builder.Services.AddAuthorization();
// Learn more about configuring OpenAPI at https://aka.ms/aspnet/openapi
builder.Services.AddOpenApi();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
}
app.UseHttpsRedirection();
//4.jwt
app.UseAuthentication();
app.UseAuthorization();
app.MapControllers();
app.Run();
Appsettings.json
- JwtSettings is added as part of JWT settings
- Secret key has to be kept not in this json, but somewhere else where it is masked.
{
"JwtSettings": {
"Issuer": "https://joydipkanjilal.com/",
"Audience": "https://joydipkanjilal.com/",
"SecretKey": "this is my custom Secret key for authentication",
"ExpiryMinutes": 60
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
https://localhost:7290/api/Auth/token
- This gives you the bearer token. The bearer token something looks like this as per below.
- The below token will be used in the CompanyController to do the Get call where it is decorated with authorize attribute.
- https://localhost:7290/api/Company
- you add the bearertoken in authorization. you can see the screenshot below.
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJ0ZXN0dXNlciIsImp0aSI6InRlc3RwYXNzd29yZCIsImV4cCI6MTc0MDMzNzEzMSwiaXNzIjoiaHR0cHM6Ly9qb3lkaXBrYW5qaWxhbC5jb20vIiwiYXVkIjoiaHR0cHM6Ly9qb3lkaXBrYW5qaWxhbC5jb20vIn0.Vkx-4Dw-fxjK4EBX8ZOq6OEb_aoxgMM_L8akgddr0HA
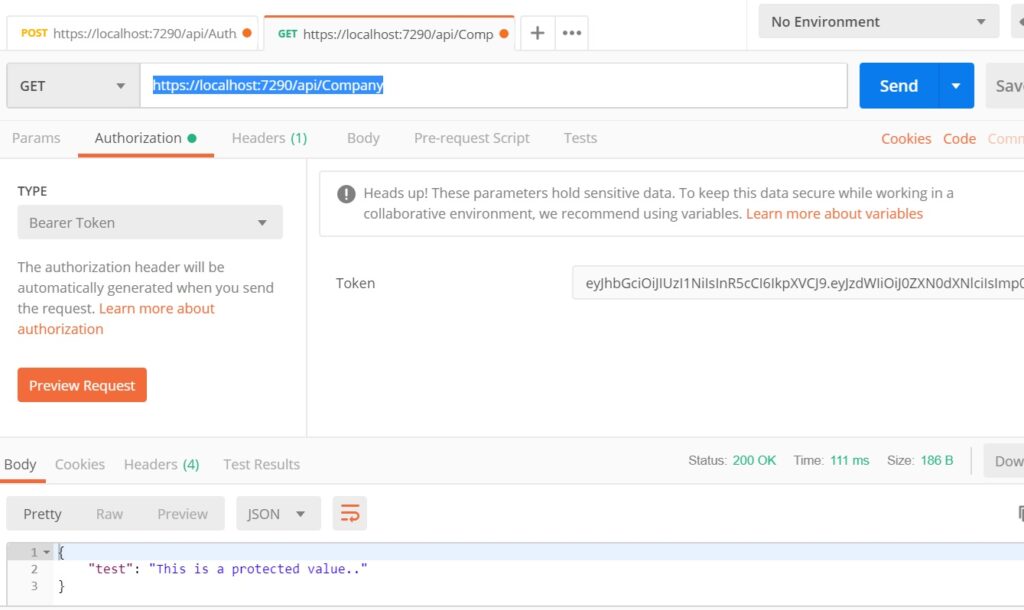