- Behavioral pattern
- The intent of this pattern is to capture and externalize and objects internal state so that the object can be restored to this state later, without violating encapsulation.
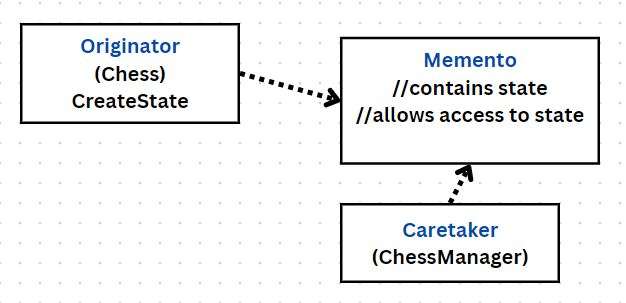
using Newtonsoft.Json;
namespace memento
{
//Originator
class Chess
{
public string? Move;
public Memento CreateState()
{
return new Memento(Move!);
}
public void Undo(Memento memento)
{
Move = memento.Move;
}
}
//Memento
public class Memento
{
public string Move { get { return move; } }
private string ? move;
public Memento(string move)
{
this.move = move;
}
}
//Creator
class ChessManager
{
Stack mementos = new Stack();
public void AddMememento(Memento state)
{
mementos.Push(state);
}
public Memento GetMemento()
{
return mementos.Pop();
}
}
}
PROGRAM.CS
// See https://aka.ms/new-console-template for more information
using memento;
var chess = new Chess() { Move = "Horse Moves L step"};
var chessManager = new ChessManager();
chessManager.AddMememento(chess.CreateState());
Console.WriteLine(chess.Move);
chess.Move = "soldier moves 1 step";
chessManager.AddMememento(chess.CreateState());
Console.WriteLine(chess.Move);
chess.Move = "Queen moves";
chessManager.AddMememento(chess.CreateState());
Console.WriteLine(chess.Move);
Console.WriteLine("-------------------------");
chess.Undo(chessManager.GetMemento());
chess.Undo(chessManager.GetMemento());
Console.WriteLine(chess.Move);
chess.Undo(chessManager.GetMemento());
Console.WriteLine(chess.Move);
OUTPUT
Horse Moves L step
soldier moves 1 step
Queen moves
————————-
soldier moves 1 step
Horse Moves L step
GITHUB