Program.cs
- IDiscount is new interface
- CouponApplyMBBSCoachingDiscount : IDiscount
- StateWiseMBBSCoachingDiscount : IDiscount
- We create one more set of DiscountFactory…
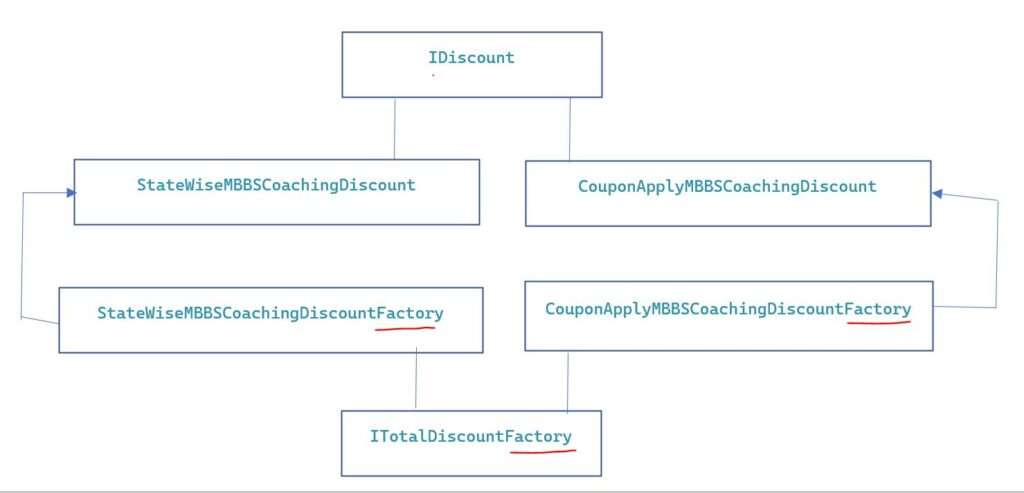
namespace simple_factory
{
interface ITotalCost
{
decimal TotalCost { get; }
}
internal class StateWiseMBBSCoachingCost : ITotalCost
{
private readonly string _stateCode;
public StateWiseMBBSCoachingCost(string stateCode)
{
_stateCode = stateCode;
}
public decimal TotalCost
{
get
{
switch (_stateCode)
{
case "WB":
return 25500;
case "MP":
return 35500;
case "Goa":
return 15500;
default:
return 20000;
}
}
}
internal class CouponApplyMBBSCoachingCost : ITotalCost
{
private readonly Guid _couponCode;
public CouponApplyMBBSCoachingCost(Guid couponCode)
{
_couponCode = couponCode;
}
public decimal TotalCost
{
get
{
//check for cup
return 5000;
}
}
}
public interface ITotalCostFactory
{
public ITotalCost CreateTotalCostService();
}
internal class CouponApplyMBBSCoachingCostFactory : ITotalCostFactory
{
private readonly Guid _couponCode;
public CouponApplyMBBSCoachingCostFactory(Guid couponCode)
{
_couponCode = couponCode;
}
public ITotalCost CreateTotalCostService()
{
return new CouponApplyMBBSCoachingCost(_couponCode);
}
}
internal class StateWiseMBBSCoachingCostFactory : ITotalCostFactory
{
private readonly string _stateCode;
public StateWiseMBBSCoachingCostFactory(string stateCode)
{
_stateCode = stateCode;
}
public ITotalCost CreateTotalCostService()
{
return new StateWiseMBBSCoachingCost(_stateCode);
}
}
}
}
Added newly
namespace simple_factory
{
public interface IDiscount
{
decimal Discount { get; }
}
internal class StateWiseMBBSCoachingDiscount : IDiscount
{
public decimal Discount => 2000;
}
//added newly
internal class CouponApplyMBBSCoachingDiscount : IDiscount
{
public decimal Discount => 1000;
}
public interface ITotalDiscountFactory
{
public IDiscount CreateTotalDiscountService();
}
public class CouponApplyMBBSCoachingDiscountFactory : ITotalDiscountFactory
{
public IDiscount CreateTotalDiscountService()
{
return new CouponApplyMBBSCoachingDiscount();
}
}
internal class StateWiseMBBSCoachingDiscountFactory : ITotalDiscountFactory
{
public IDiscount CreateTotalDiscountService()
{
return new StateWiseMBBSCoachingDiscount();
}
}
}
Program.cs
var ddownValue = "WB";
ITotalCostFactory factoryObj1;
ITotalDiscountFactory factoryObj2;
factoryObj1 = new CouponApplyMBBSCoachingCostFactory(new Guid());
factoryObj2 = new CouponApplyMBBSCoachingDiscountFactory();
Console.WriteLine(factoryObj1.CreateTotalCostService().TotalCost - factoryObj2.CreateTotalDiscountService().Discount);
OUTPUT
4000
(50000-1000)
- Next post is on Abstract factory pattern
- The same output we will get using the abstract factory pattern..